Kudosity SMS and Webhook Essentials
We use Glitch.com to show how to integrate with Kudosity. We believe that working code beats videos, long-winded tutorials, complicated diagrams, etc, every day of the week. Please Remix, Share, Chat, Post, etc, about our code and help us spread the love and improve our services. Keep in mind, this is just sharable sample code and not production ready.
This tutorial covers
- Sending an SMS through api.transmitsms.com
- Setting up a webhook in Kudosity
- Receiving the SMS webhook and displaying it on the page
Setup
- Sign up: Register for a free Kudosity developer account: Kudosity Developer Account
- Remix Project: Remix the Glitch project sms-kudosity-webhook-example.
- Configure Webhooks: Set the DLR Callback URL in your Kudosity account to:
yourproject.glitch.me/message
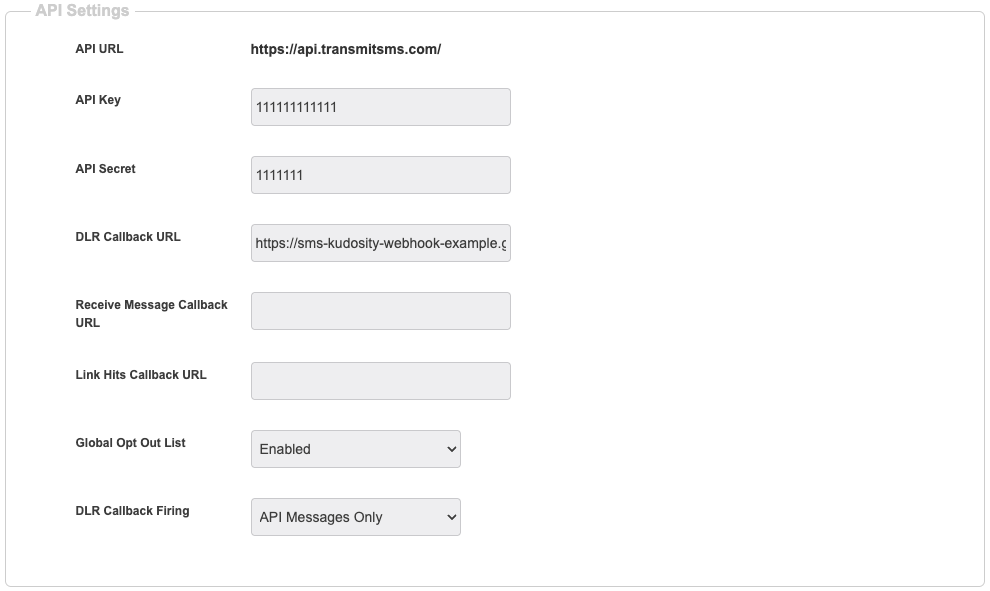
-
Run the project:
- Fill in the form details and click send
- TO: Only numbers in your “My Test List” can be used. Read more about Kudosity Trial Limitations.
- API_KEY + API Secret: https://kudosity.transmitsms.com/u/settings/api
Workflow Overview
Once you send the SMS:
- The project sends and SMS through
api.transmitsms.com
- The SMS is delivered to the recipient.
- The telco provider sends a delivery status update to Kudosity.
- Kudosity forwards the status as a webhook to your project:
yourproject.glitch.me/message
- The project displays the webhook on the page.
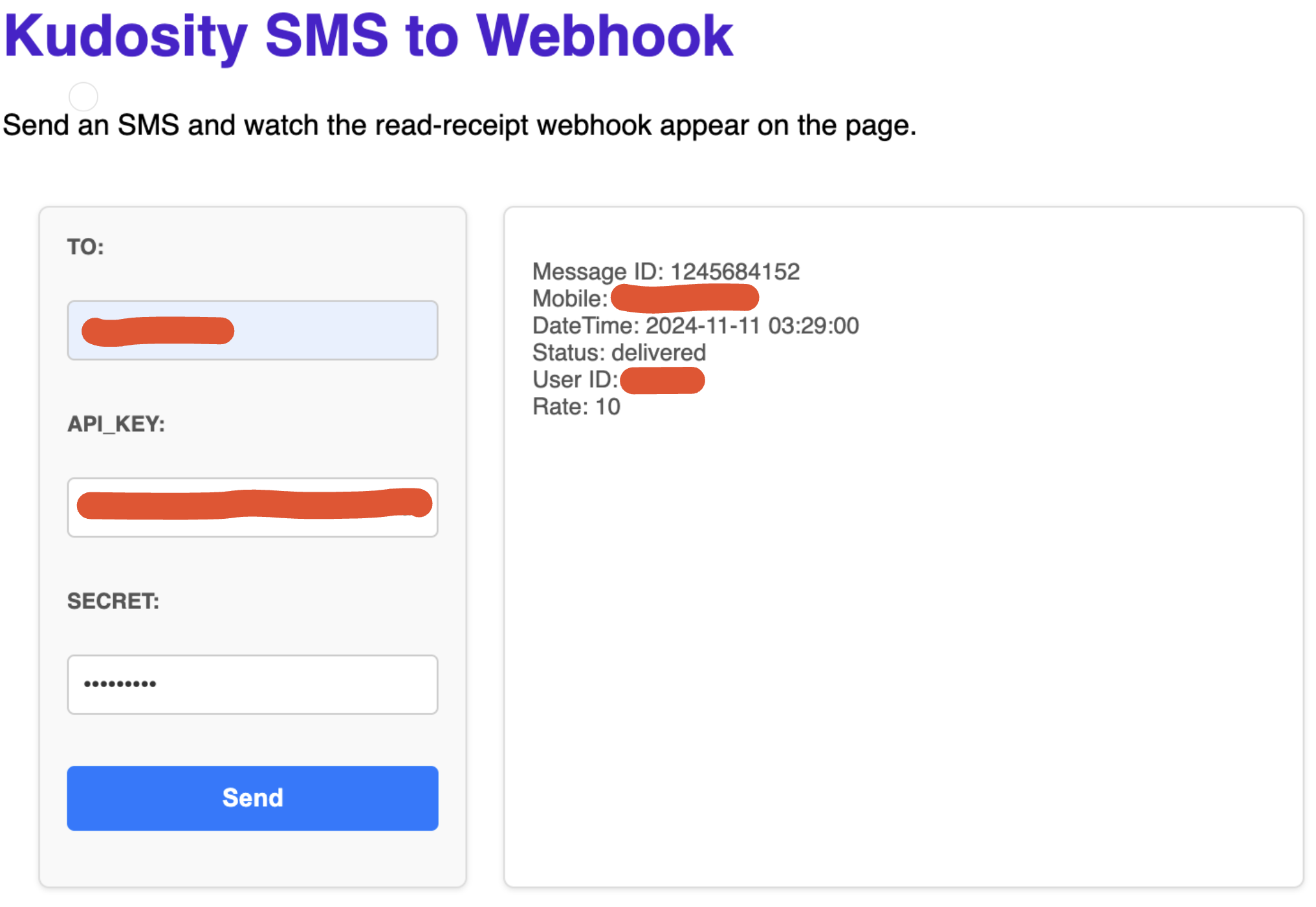
Key Points About the Code
Below are salient points about the code. Keep in mind this is just sample code for illustration purposes. How you implement this on your production environment may differ.
Sending SMS
Endpoint: /sendmessage
This POST route processes form data and sends a request to the TransmitSMS API.
- Authorization: Credentials (API_KEY and SECRET) are base64-encoded for Basic Authentication.
- Request Preparation: Form data is appended using
URLSearchParams
. - Request Method: The
fetch
method sends a POST request to the api.
app.post('/sendmessage', async (req, res) => {
const to = req.body.to;
const apiKey = req.body.api_key;
const secret = req.body.secret;
const credentials = `${apiKey}:${secret}`;
const base64Credentials = Buffer.from(credentials, 'ascii').toString('base64');
const url = 'https://api.transmitsms.com/send-sms.json';
const data = new URLSearchParams();
data.append('message', 'Hello from Kudosity');
data.append('to', to);
const fetch = (await import('node-fetch')).default;
try {
const response = await fetch(url, {
method: 'POST',
headers: {
'Authorization': `Basic ${base64Credentials}`,
'Content-Type': 'application/x-www-form-urlencoded'
},
body: data
});
const result = await response.json();
console.log('SMS API response:', result);
} catch (error) {
console.error('Error sending SMS:', error);
res.status(500).json({ error: 'Failed to send SMS' });
}
res.sendStatus(204);
});
Webhook Integration
Endpoint: /message
The webhook endpoint receives GET requests from TransmitSMS. Key parameters such as message_id, mobile, datetime, status, user_id, and rate are extracted and stored in a global message object.
app.get("/message", function (request, response) {
message['message_id'] = request.query.message_id;
message['mobile'] = request.query.mobile;
message['datetime'] = request.query.datetime;
message['status'] = request.query.status;
message['user_id'] = request.query.user_id;
message['rate'] = request.query.rate;
response.sendStatus(200);
});
Integration Notes
Webhook Registration: Configure the callback URL (/message) in your TransmitSMS API Settings.
Security: Add signature verification to validate the webhook's authenticity. Learn more: Validating Webhook Signatures from Kudosity.
Updated 6 days ago